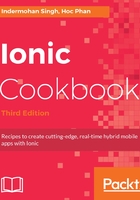
上QQ阅读APP看书,第一时间看更新
How to do it...
The following are the instructions to create example app:
- Create a new PagesAndTabs app using the tabs template and go into the PagesAndTabs folder to start Visual Studio Code, as shown:
$ ionic start PagesAndTabs tabs $ cd PagesAndTabs $ code .
- The blank template only gives you a basic page. Open the Finder app in Mac or Windows Explorer in Windows to see the following folder structure:

You will only modify what is in the /src folder and not /www, as in Ionic 1. Everything in the /src folder will be built and the /www folder will be created automatically. We will also reserve the folder names and filenames as much as possible, since the main goal here is to understand how the tab template works and the areas you can modify.
- Open and edit the /src/pages/tabs/tabs.html template file with the
following code:
<ion-tabs> <ion-tab [root]="tab1Root" tabTitle="One"
tabIcon="water"></ion-tab> <ion-tab [root]="tab2Root"
tabTitle="Two"
tabIcon="leaf"></ion-tab> <ion-tab [root]="tab3Root"
tabTitle="Three"
tabIcon="flame"></ion-tab> </ion-tabs>
The new template only updates the title and icons. This is because this example wants to reserve the naming of the tab root variables. You could add more tabs using <ion-tab>, as needed.
- To add a page, you need to ensure that tab1Root points to an existing folder and template. Since you will reuse the existing tab structure, you can just modify the /src/pages/home/home.html template, as shown, as this is your first page:
<ion-header>
<ion-navbar>
<ion-title>One</ion-title>
</ion-navbar>
</ion-header>
<ion-content padding>
<h2>Welcome to Ionic!</h2>
<p>
This starter project comes with simple tabs-based layout for
apps
that are going to primarily use a Tabbed UI.
</p>
<p>
Take a look at the <code>src/pages/</code> directory to add or
change tabs, update any existing page or create new pages.
</p>
</ion-content>
- Also, in the same /home folder, edit home.ts, which corresponds to the same template, and enter the code here:
import { Component } from '@angular/core';
import { NavController } from 'ionic-angular';
@Component({
selector: 'page-home',
templateUrl: 'home.html'
})
export class HomePage {
constructor(public navCtrl: NavController) {
}
}
- For the second page, tab2Root, you will follow a similar process by editing the /src/pages/about/about.html template as shown:
<ion-header>
<ion-navbar>
<ion-title>
Two
</ion-title>
</ion-navbar>
</ion-header>
<ion-content>
<ion-list>
<ion-item>
<ion-input type="text" placeholder="First Name"></ion-input>
</ion-item>
<ion-item>
<ion-input type="text" placeholder="Last Name"></ion-input>
</ion-item>
<div padding>
<button ion-button primary block>Create Account</button>
</div>
</ion-list>
</ion-content>
- Edit about.ts in the same folder as in the preceding step:
import { Component } from '@angular/core';
import { NavController } from 'ionic-angular';
@Component({
selector: 'page-about',
templateUrl: 'about.html'
})
export class AboutPage {
constructor(public navCtrl: NavController) {
}
}
- Finally, for the tab3Root page, you can change the template so that it will show a slider in /src/pages/contact/contact.html, as follows:
<ion-header>
<ion-navbar>
<ion-title>
Three
</ion-title>
</ion-navbar>
</ion-header>
<ion-content>
<ion-slides #mySlider index=0 (ionSlideDidChange)="onSlideChanged($event)">
<ion-slide style="background-color: green">
<h2>Slide 1</h2>
</ion-slide>
<ion-slide style="background-color: blue">
<h2>Slide 2</h2>
</ion-slide>
<ion-slide style="background-color: red">
<h2>Slide 3</h2>
</ion-slide>
</ion-slides>
</ion-content>
- In the /contact folder, you need to edit contact.ts with the following code:
import { Component, ViewChild } from '@angular/core';
import { NavController, Slides } from 'ionic-angular';
@Component({
selector: 'page-contact',
templateUrl: 'contact.html'
})
export class ContactPage {
@ViewChild('mySlider') slider: Slides;
constructor(public navCtrl: NavController) {
}
onSlideChanged(e) {
let currentIndex = this.slider.getActiveIndex();
console.log("You are on Slide ", (currentIndex + 1));
}
}
- Go to your Terminal and type the following command line to run the app:
$ ionic serve