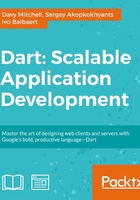
Handout notes
There is nothing like a tangible handout to give presentation attendees. This can be achieved with a variation of the overview display:

Instead of duplicating the overview code, the function can be parameterized with an optional parameter in the method declaration. This is declared with square brackets []
around the declaration and a default value that is used if no parameter is specified:
void buildOverview([bool addNotes = false])
This is called by the presentation overview display without requiring any parameters:
buildOverview();
This is called by the handouts display without requiring any parameters:
buildOverview(true);
If this parameter is set, an additional Div
element is added for the Notes
area and the CSS is adjusted for the benefit of the print layout.
Comparing optional positional and named parameters
The addNotes
parameter is declared as an optional positional parameter, so an optional value can be specified without naming the parameter. The first parameter is matched to the supplied value.
To give more flexibility, Dart allows optional parameters to be named. Consider two functions—the first will take named optional parameters and the second will take positional optional parameters:
getRecords1(String query,{int limit: 25, int timeOut: 30}) { } getRecords2(String query,[int limit = 80, int timeOut = 99]) { }
The first function can be called in more ways:
getRecords1(""); getRecords1("", limit:50, timeOut:40); getRecords1("", timeOut:40, limit:65); getRecords1("", limit:50); getRecords1("", timeOut:40); getRecords2(""); getRecords2("", 90); getRecords2("", 90, 50);
With named optional parameters, the order they are supplied in is not important and has the advantage that the calling code is clearer as to the use that will be made of the parameters being passed.
With positional optional parameters, we can omit the later parameters, but it works in a strict left-to-right order, so to set the timeOut
parameter to a non-default value, the limit must also be supplied. It is also easier to confuse which parameter is for which particular purpose.