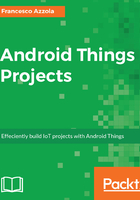
How to use GPIO pins
When we connect peripherals to an Android Things board we use pins. There several types of pins. This project uses GPIO pins. GPIO stands for General Purpose Input Output. These pins are the interface between the board (such as Raspberry or Intel Edison) and the world. You can think of them as a switch that can be turned on or off. Using GPIO pins we handle binary devices. A GPIO pin can have only two states:
- On or High level
- Off or Low or zero
According to the nature of these pins, we can connect to these pins all the peripherals that have two states. Typical examples of these peripherals are switches or simple LEDs (only one color led). The PIR sensor described previously belongs to this category.
Android Things SDK provides an important class that helps us to interact with GPIO pins hiding the communication details. This class is called PeripheralManagerService. Using PeripheralManagerService we can do several actions on the pins:
- Get the pins list
- Get the pin state
- Set the pin state
These are the main actions; anyway, this class provides several methods that help us to manage the pin connection and its state.
To use a GPIO pin in Android Things we have to follow three steps:
- Get an instance of PeripheralManagerService.
- Open the connection to the pin using the pin identifier.
- Declare if the pin is used to read (input) or to write (output).
Let us see how we can implement it in our project.
Clone the Android Things project template as described in the previous chapter. Open MainActivity.java and in the onCreate method add the following:
PeripheralManagerService service = new PeripheralManagerService();
In this way, we get an instance of PeripheralManagerService, the class that handles the GPIO communication details.
In the onCreate method add the following lines:
try {
gpioPin = service.openGpio(GPIO_PIN);
gpioPin.setDirection(Gpio.DIRECTION_IN);
gpioPin.setActiveType(Gpio.ACTIVE_HIGH);
}
catch(IOexception ioe) {}
The preceding code is simple: the app opens a connection to a GPIO pin specified in GPIO_PIN. By now you should remember that Raspberry Pi 3 and Intel Edison have a different GPIO pinout. According to the schema shown previously, the pin names are:
- BMC 4 for Raspberry
- IO4 for Intel Edison with Arduino breakout kit
After that, the app specifies the type of connection it will handle. In this project, we want to read from the pin so we declare Gpio.DIRECTION_IN. As we said before, a GPIO pin can be used to read or write so there are two possible values:
- Gpio.DIRECTION_IN if we read
- Gpio.DIRECTION_OUT if we write
Finally, we have to set if we want that true value corresponding to the high voltage level or zero level. We do it using setActiveType that accepts two values:
- Gpio.ACTIVE_HIGH: The value is true if the pin is at high voltage
- Gpio.ACTIVE_LOW: The value is true if the pin is at low voltage or zero
All these methods can raise an IOException when a problem occurs. For this reason, they are in a try/catch clause.