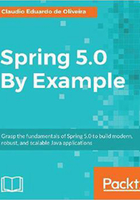
First documented API
We will start with the CategoryResource class, because it is simple to understand, and we need to keep the focus on the technology stuff. We will add a couple of annotations, and the magic will happen, let's do magic.
The CategoryResource class should look like this:
package springfive.cms.domain.resources;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiResponse;
import io.swagger.annotations.ApiResponses;
import java.util.List;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.RestController;
import springfive.cms.domain.models.Category;
import springfive.cms.domain.service.CategoryService;
import springfive.cms.domain.vo.CategoryRequest;
@RestController
@RequestMapping("/api/category")
@Api(tags = "category", description = "Category API")
public class CategoryResource {
private final CategoryService categoryService;
public CategoryResource(CategoryService categoryService) {
this.categoryService = categoryService;
}
@GetMapping(value = "/{id}")
@ApiOperation(value = "Find category",notes = "Find the Category by ID")
@ApiResponses(value = {
@ApiResponse(code = 200,message = "Category found"),
@ApiResponse(code = 404,message = "Category not found"),
})
public ResponseEntity<Category> findOne(@PathVariable("id") String id){
return ResponseEntity.ok(new Category());
}
@GetMapping
@ApiOperation(value = "List categories",notes = "List all categories")
@ApiResponses(value = {
@ApiResponse(code = 200,message = "Categories found"),
@ApiResponse(code = 404,message = "Category not found")
})
public ResponseEntity<List<Category>> findAll(){
return ResponseEntity.ok(this.categoryService.findAll());
}
@PostMapping
@ApiOperation(value = "Create category",notes = "It permits to create a new category")
@ApiResponses(value = {
@ApiResponse(code = 201,message = "Category created successfully"),
@ApiResponse(code = 400,message = "Invalid request")
})
public ResponseEntity<Category> newCategory(@RequestBody CategoryRequest category){
return new ResponseEntity<>(this.categoryService.create(category), HttpStatus.CREATED);
}
@DeleteMapping("/{id}")
@ResponseStatus(HttpStatus.NO_CONTENT)
@ApiOperation(value = "Remove category",notes = "It permits to remove a category")
@ApiResponses(value = {
@ApiResponse(code = 200,message = "Category removed successfully"),
@ApiResponse(code = 404,message = "Category not found")
})
public void removeCategory(@PathVariable("id") String id){
}
@PutMapping("/{id}")
@ResponseStatus(HttpStatus.NO_CONTENT)
@ApiOperation(value = "Update category",notes = "It permits to update a category")
@ApiResponses(value = {
@ApiResponse(code = 200,message = "Category update successfully"),
@ApiResponse(code = 404,message = "Category not found"),
@ApiResponse(code = 400,message = "Invalid request")
})
public ResponseEntity<Category> updateCategory(@PathVariable("id") String id,CategoryRequest category){
return new ResponseEntity<>(new Category(), HttpStatus.OK);
}
}
There are a lot of new annotations to understand. The @Api is the root annotation which configures this class as a Swagger resource. There are many configurations, but we will use the tags and description, as they are enough.
The @ApiOperation describes an operation in our API, in general against the requested path. The value attribute is regarding as the summary field on Swagger, it is a brief of the operation, and notes is a description of an operation (more detailed content).
The last one is the @ApiResponse which enables developers to describe the responses of an operation. Usually, they want to configure the status codes and message to describe the result of an operation.
Now, we have configured the Swagger integration, we can check the API documentation on the web browser. To do it, we need to navigate to http://localhost:8080/swagger-ui.html and this page should be displayed:

We can see APIs endpoints configured in our CMS application. Now, we will take a look at category which we have configured previously, click on the Show/Hide link. The output should be:

As we can see, there are five operations in our Category API, the operation has a path and a summary to help understand the purpose. We can click on the requested operation and see detailed information about the operation. Let's do it, click on List categories to see detailed documentation. The page looks like this:

Outstanding job. Now we have an amazing API with excellent documentation. Well done.
Let's continue creating our CMS application.