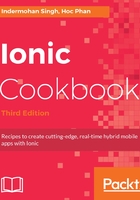
How it works...
Since this app is just an introduction to menu navigation, it will not manage page routing and state parameters. At a higher level, this is how the app flows:
- app.ts loads both of the menu templates in app.html.
- The left menu will trigger the openPage() function to open PageTwo.
- The right menu will trigger the rightMenuClick() function to change the this.text property and be displayed on the screen.
In the app.html template, the left menu has the following properties:
side="left" type="overlay"
However, the right menu has the following assigned instead:
side="right" type="reveal"
The side property will determine where on the screen the menu should show. There are two types of menus. The overlay option will leave the center page as it is, without moving. The reveal option will push the entire screen to show the menu. Which type you pick depends on the design of your app.
Each ion-menu directive must have [content]="content" declared because it will use the content area to bind swipe left or right. In this case, it is basically a local variable in ion-nav, as follows:
<ion-nav id="nav" [root]="rootPage" #content swipeBackEnabled="false"></ion-nav>
The use of ion-toolbar inside ion-menu is optional if you want to have the title for your menu. The key to having a menu item displayed is to use ion-list and ion-item. You can loop through an array to display the menu items dynamically, as illustrated:
<ion-list> <button menuClose ion-item *ngFor="let p of pages"
(click)="openPage(p)"> {{p.title}} </button> </ion-list>
*ngFor is a replacement for ng-repeat in Ionic 1. You need to use let p because it's the same as declaring a local variable named p. This is best practice for variable isolation. Otherwise, the concept is very similar to Ionic 1, as you can grab p.title for each item in the pages array.
On the right menu, instead of going to a different page via nav.setRoot(), you just set some text and dynamically display the text inside the menu, as shown:
<ion-card *ngIf="text"> <ion-card-content> You just clicked {{ text }} </ion-card-content> </ion-card>
So, if the text variable doesn't exist (which means that the user has not clicked on anything yet), the ion-card will not show anything via *ngIf.
For each page, you have to declare the same ion-navbar. Otherwise, you will lose the top navigation and buttons to the menus:
<ion-header> <ion-navbar> <ion-title>Getting Started</ion-title> <ion-buttons start> <button ion-button menuToggle="leftMenu"> <ion-icon name="menu"></ion-icon> </button> </ion-buttons> <ion-buttons end> <button ion-button menuToggle="rightMenu"> <ion-icon name="menu"></ion-icon> </button> </ion-buttons> </ion-navbar> </ion-header>
Note that leftMenu and rightMenu must be the same id you used earlier, in the app.html template.
On the first page, there are two buttons to trigger the menus from within the content page as well, as shown:
<ion-row> <ion-col width-50> <button primary block menuToggle="leftMenu">Toggle
Left</button> </ion-col> <ion-col width-50> <button primary block menuToggle="rightMenu">Toggle
Right</button> </ion-col> </ion-row>
These two buttons also call menuToggle to trigger the menu. The buttons are placed within the Ionic grid system. Since Ionic uses Flexbox, it is very simple to use—you just need to create ion-col and ion-row. The width property, with a number, will determine the width percentage.