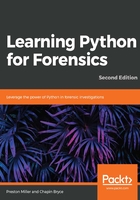
Enhancing the print_output() function
In this function, we have made some adjustments to improve the output to the console. With the addition of the separator defined on 178, we now have a line of 15 dashes visually breaking each entry from the output. As we can see, we have borrowed the same format string from the first iteration to add this break:
171 def print_output(usb_information):
172 """
173 Print formatted information about USB Device
174 :param usb_information: dictionary containing key/value
175 data about each device or tuple of device information
176 :return: None
177 """
178 print('{:-^15}'.format(''))
We have also modified the code to allow additional output for flexible fields. In this function, we need to handle two different data types, tuples and dictionaries, since some entries do not have a resolved vendor or product name. To handle this divide in formats, we must use the isinstance() function on line 180 to test the usb_information variable data type. If the value is a dictionary, we will print each of the keys and values to the console to display one key-value pair per line on line 182. This is possible through the combination of the for loop on line 181, which uses the items() method on a dictionary. This method returns a list of tuples, where the first tuple element is the key and the second is the value. Using this method, we can quickly extract the key-value pairs, as shown on lines 181 and 182:
180 if isinstance(usb_information, dict):
181 for key_name, value_name in usb_information.items():
182 print('{}: {}'.format(key_name, value_name))
If we need to print a tuple, we use two print statements, similar to the output from the prior iteration. Because this data is from a device that could not be parsed, it has a fixed format that is the same as our previous iteration. See the following lines:
183 elif isinstance(usb_information, tuple):
184 print('Device: {}'.format(usb_information[0]))
185 print('Date: {}'.format(usb_information[1]))