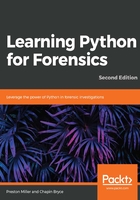
Troubleshooting
At some point in your development career—probably by the time you write your first script—you will have encountered a Python error and received a Traceback message. A Traceback provides the context of the error and pinpoints the line that caused the issue. The issue itself is described as an exception, and usually provides a human-friendly message of the error.
Python has a number of built-in exceptions, the purpose of which is to help the developer in diagnosing errors in their code. A full listing of built-in exceptions can be found at https://docs.python.org/3/library/exceptions.html.
Let's look at a simple example of an exception, AttributeError, and what the Traceback looks like in this case:
>>> import math
>>> print(math.noattribute(5))
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'module' object has no attribute 'noattribute'
The Traceback indicates the file in which the error occurred, in this case, stdin or standard input, because this code was written in the interactive prompt. When working on larger projects or with a single script, the file will be the name of the script causing the error rather than stdin. The in <module> bit will be the name of the function that contains the faulty line of code, or <module> if the code is outside of a function.
Now, let's look at a slightly more complicated issue. To do this, let's use the data structure from our prior script. In the following code block, we are not accessing the VID data with the get() call, but instead hoping that it exists. Temporarily replace line 66 of the usb_lookup.py script with the following for this example:
066 vendor = usb_dict[vendor_key]
Now, if you run this updated code with a valid vendor key, you will get an expected result, though use a key such as ffff and see what occurs. Check if it looks like the following:
$ python usb_lookup.py ffff 1643
Traceback (most recent call last):
File "usb_lookup.py", line 90, in <module>
main(args.vid, args.pid)
File "usb_lookup.py", line 62, in main
search_key(vid, pid, usbs)
File "usb_lookup.py", line 66, in search_key
vendor = usb_dict[vendor_key]
KeyError: 'ffff'
The traceback here has three traces in the stack. The last trace at the bottom is where our error occurred. In this case, on line 66 of the usb_lookup.py file, the search_key() function generated a KeyError exception. Looking up what a KeyError exception is in the Python documentation would indicate that this is due to the key not existing in the dictionary. Most of the time, we will need to address the error at that specific error causing line. In our case, we employed the get() method of a dictionary to safely access key elements. Please revert the line back to its prior state at this time to prevent this error from occurring in the future!