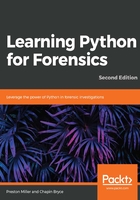
Interpreting the search_key() function
The search_key() function, originally called on line 62 of the main() function, is where we search for the user-supplied vendor and product IDs, and display the resolved results to the user. In addition, all of our error handling logic is contained within this function.
Let's practice accessing nested lists or dictionaries. We discussed this in the main() function; however, it pays to actually practice rather than take our word for it. Accessing nested structures requires us to use multiple indices rather than just one. For example, let's create a list and map that to key_1 in a dictionary. To access elements from the nested list, we will need to supply key_1 to access the list and then a numerical index to access elements of the list:
>>> inner_list = ['a', 'b', 'c', 'd']
>>> print(inner_list[0])
a
>>> outer_dict = {'key_1': inner_list}
>>> print(outer_dict['key_1'])
['a', 'b', 'c', 'd']
>>> print(outer_dict['key_1'][3])
d
Now, let's switch gears, back to the task at hand, and leverage our new skills to search our dictionary to find vendor and product IDs. The search_key() function is defined on line 65 and takes the user-supplied VID and PID along with our parsed out usb_dict dictionary. We then start by querying usb_dict for the vendor_key value, using the get() method of a dictionary to attempt to get the requested key's value or return None, as specified on line 66, if the key is not found.
Please note that the data returned by the get() call, if successful, is the entire value for that key, or in this case a list, where element zero is the vendor name and element one is the dictionary of product details. We can then check, on line 67, to see whether the key was found; if it was unavailable, we print this to the user and exit on lines 68 and 69, as shown here:
065 def search_key(vendor_key, product_key, usb_dict):
066 vendor = usb_dict.get(vendor_key, None)
067 if vendor is None:
068 print('Vendor ID not found')
069 exit()
We can then repeat this logic for looking up the product information, though we first have to navigate to the product information. On line 71, we access element one of the vendor list, containing the product details dictionary, and perform the same get() method call to look up any name resolution for the PID. In the same manner, we check to see if the lookup failed and provide any available details to the user; in case it fails, we can at least give the vendor information:
071 product = vendor[1].get(product_key, None)
072 if product is None:
073 print('Vendor: {}\nProduct Id not found.'.format(
074 vendor[0]))
075 exit(0)
If everything resolves successfully, we can print the output to the user and the script will complete! Notice how, on line 77, in the format statement, we have to call the first element of the vendor variable since the value of the VID key lookup was a list, whereas the value of the PID key lookup is just the product's name. This is where things can get a little confusing, though feel free to reference the earlier sample data structure and add as many intermediate print statements to help with comprehension:
077 print('Vendor: {}\nProduct: {}'.format(vendor[0], product))